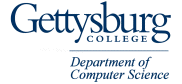 |
CS 107 - Introduction to Scientific Computation Homework #11 |
Due: Wednesday 11/20 at the beginning of class
Object-Oriented Debugging*
In this simulation assignment, you will create a non-grid-based simulation of
bugs moving in a constrained area among traps, plotting simulation states,
and tracking the population decline. While significantly different, this
assignment is based on the insect trapping research work of John A. Byers:
0. Preparatory Reading: You'll want to feel comfortable with creating
arrays of Object, so the Matlab User Guide on
Creating Object Arrays is recommended.
1. Bug: Create a Bug handle class according to these
specifications:
- Properties:
- moveSD: standard deviation of random normal distribution for movement
distance, default value .05
- x: current bug x position, 0 <= x < 1
- y: current bug y position, 0 <= y < 1
- Methods:
- Function Bug (constructor)
- return value: self
- parameter 1: moveSD: user-defined standard deviation of random normal
distribution for movement distance
- if nargin > 0, parameter 1 is copied to property moveSD
- properties x and y are assigned random numbers, 0 <= x < 1, 0 <= y
< 1
- Function move
- no return value
- parameter 1: self
- Compute a random movement distance according to a normal distribution
with mean 0 and standard deviation moveSD.
- Compute a random angle according to a uniform distribution over all
possible angles.
- Convert distance and angle to change in x and y position, and make that
change.
- If the Bug moves beyond the unit square with opposite corners (0, 0) and
(1, 1), treat the Bug as if it perfectly bounces off the sides of the
constrained space.
2. Trap: Create a Trap handle class according to these
specifications:
- Properties:
- catchRadius: the distance from the Trap within which a Bug is trapped,
default value .05
- x: Trap x position, 0 <= x < 1
- y: Trap y position, 0 <= y < 1
- Methods:
- Function Trap (constructor)
- return value: self
- parameter 1: catchRadius: user-defined distance from the Trap within
which a Bug is trapped
- if nargin > 0, parameter 1 is copied to property catchRadius
- properties x and y are assigned random numbers, 0 <= x < 1, 0 <= y
< 1
- Function canCatch
- return value: can: a Boolean (true/false) value indicating whether or
not a Bug is within the Trap's catch radius
- parameter 1: self
- parameter 2: bug: a Bug
- Compute the distance between the Trap and Bug positions, and return
whether or not this is <= catchRadius.
3. BugWorld: Create a BugWorld handle class according to these
specifications:
- Properties:
- bugs: an array of Bug objects in the BugWorld, initially a single
default (no-argument) Bug
- traps: an array of Trap objects in the BugWorld, initially a single
default (no-argument) Trap
- Methods:
- Function BugWorld (constructor)
- return value: self
- parameter 1: numBugs: the initial number of Bug objects in
BugWorld
- parameter 2: moveSD: user-defined standard deviation of random
normal distribution for movement distance for each Bug
- parameter 3: numTraps: the initial number of Trap objects in
BugWorld
- parameter 4: catchRadius: user-defined distance from each Trap
within which a Bug is trapped
- Create the given number of Bug and Trap objects in the bugs and
traps array properties according to the given constructor arguments.
- Function tick:
- return value: self
- parameter 1: self
- For each bug in bugs,
- call the Bug move method
- check each Trap to see if it catches the Bug
- if a Trap catches the Bug, remove it from the bugs array
- Function ticks:
- return value: bugNums: an array of bug population values before
and after each successive simulation tick (step)
- parameter 1: self
- parameter 2: numTicks: a given number of simulation ticks
(steps)
- Add the current Bug population to bugNums.
- Perform the given number of simulation ticks, adding the new
population to bugNums after each tick.
- Function plotWorld:
- return value: (none)
- parameter 1: self
- Clear current axes with command "cla", and turn hold on.
- Create a square axis from (0, 0) to (1, 1)
- Create two arrays of bug x and y values, respectively, and plot
them using the "scatter" command.
- For each trap, draw a circle according to the cach radius.
- Turn hold off.
You may find the following sets of test commands helpful. To visually
test the step-by-step simulation:
>> clear
>> w = BugWorld(100, .05, 10, .05);
>> w.tick(); w.plotWorld();
>> w.tick(); w.plotWorld();
... (repeat) ...
One can comment out the plotWorld "cla" command to keep previous Bug positions on the plot.
To plot the population trend over successive simulations:
>> hold on;
>> w = BugWorld(100, .05, 10, .05);
>> plot(w.ticks(1000))
>> w = BugWorld(100, .05, 10, .05);
>> plot(w.ticks(1000))
... (repeat) ...
*The author understands that the original work concerned flying insects and
that "bug" and "insect" are distinct terms, but the pun was just too good to
pass up.