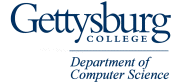 |
CS 112 - Introduction to Computer Science II
Homework #1
|
Assignment work will be individual for this assignment only. Due: at the beginning of class 3.
Include a README file with the honor pledge, your name and student ID, and
written exercises. We will cover the submission process in class.
Be sure to have your README and ModularPascalsTriangle.java in the same default package directory.
If you'd like to submit your work before Friday and get correctness feedback on
your ModularPascalsTriangle.java, this is how we'll be submitting work
together in class on Friday:
- Get your README file and your ModularPascalsTriangle.java into the same
directory. It doesn't matter which, but you'll probably find it most
convenient to submit from where you develop, e.g. ~/eclipse-workspace/cs112 .
- In your README, be sure you have your:
- Honor pledge
- Name
- Student ID number
- One paragraph summary of pair programming
- Transcript of commands entered for the bash tutorial (or 3 new
things learned beyond the tutorial)
- Open a terminal window and change to the directory with all of your
submission files, e.g. "cd ~/eclipse-workspace/cs112".
- Enter the command "submit112 hw1".
- Wait several seconds (usually not more than a minute).
- Check the generated feedback file feedback.txt using the command "less
feedback.txt". When using the "less" command, "q" quits, "enter"
advances a line, and "space" advances a page. The mouse wheel also
works.
- Note: You may submit your work up to 10 times. (Further
submissions will provide feedback, but will not be graded.) It is best to test
your work on your own before submission. Do not rely on the submission
system to serve as your testing software. Software testing is
a skill you should build in this class.
1. Bash: Have you already learned the
basics of the bash shell as represented in our
tutorial?
-
Yes: Consult online documentation, learn three new things about the bash
shell, and describe them briefly. Include these descriptions in your
README file.
-
No: Do the bash tutorial ("Getting
started with bash at Gettysburg"). Do
the tutorial in one sitting so that you'll be able to submit a transcript
of your shell commands. These will be appended to a "README" file which
can be submitted via Moodle.
2. Eclipse: (Optional: Watch the
Deitel Eclipse
introduction video. This can be viewed on non-Linux campus computers.) In
class: Do the Eclipse tutorial "Create
a Hello World application". Get to the Welcome screen either at first
startup or via Help→Welcome. Choose "Tutorials".
Under "Java Development", choose "Create
a Hello World application". Follow the tutorial instructions step-by-step in
the "Welcome" tab in the right margin. Since we'll be doing this
in class, there will be nothing you'll need to submit; your attendance and
participation will give you full credit.
Note: We will be changing one default in Eclipse. Window → Preferences
→ Java → Build Path: Select "Project" radio button for
"Source and output folder". Click "Apply and Close" button.
Do this before creating your first Java project.
The more you know of your work environment, the more efficient you will be. A little time spent above and
beyond these tutorials will likely pay off significantly in the long run as a CS
major/minor.
3. Modular Pascal's Triangle: Read about
Pascal's Triangle.
For this exercise, you will be computing a 2D array with a Modular Pascal's
Triangle, that is, a Pascal's Triangle formed with
modular addition.
(This will be similar in arrangement to the last Fibonacci pattern figure of
this
Wikipedia article section, with blank areas filled with 0's.) Implement and submit ModularPascalsTriangle.java according to
this specification.
Hint: It will be easiest if you approach this as we approached the creation
of BankAccount in class:
- Define your fields. ("What does the ModularPascalsTriangle object
'know' about itself?")
- You can auto-generate the beginning of your constructor according to the
specification (right click, "Source", "Generate Constructor using
Fields...").
- Further, you can auto-generate your getters for rows, cols, and mod
(right click, "Source", "Generate Getters and Setters...")
A
ModularPascalsTriangle video tutorial for setting up your class definition is also available. NOTE: This does NOT cover how to do the core modular Pascal's triangle computation. That's your independent work.
You'll also need to create a field for your 2D array of values,
initialization code for it in your constructor, and the specified getter method
for reading individual values from it given a row and column.
Optionally, here's some fun you can have while testing your program:
- You may visualize the beautiful patterns formed by your code using
ModularPascalsTrianglePlot.java. For modulus 2, you'll see the
Sierpinski
triangle pattern. This needs to be compiled in the same directory
as your ModularPascalsTriangle.java and should be run from the command
prompt as follows: "java ModularPascalsTrianglePlot <size> <mod>" where the
<size> is the number of rows and the number of columns. E.g.
"java ModularPascalsTrianglePlot 400 5". Zeros are colored white and
from 1 to the modulus minus 1 fades from red to yellow to green to blue.
- For any prime modulus, what do you observe about the patterns? Try
2, 3, 5, 7, 11, and 107 for different sizes.
- For these prime modulus plots, what do you observe at the top of each of the
white (zero) downward triangles?
- For larger prime modulus plots (e.g. 997, 104729), what do you observe within
the non-white (non-zero) regions?
- For simple non-primes (e.g. 6), do you observe any interesting patterns?
4. Pair Programming: In this course, you'll practice the
Agile
software development practice of Pair
Programming (described simply
here) for producing correct, high-quality solutions. In your README
file, include a single paragraph that defines "pair programming" and describes a
summary of the practice in your own words.
Rubric: (20 points total)
- 6 points: Bash
- tutorial path:
- all tutorial commands executed and evidenced in the README file
output of history command: full credit
- for every 3 tutorial commands missed, -1 point
- learn three new things (not in tutorial):
- for each piece of new bash knowledge described that is not
in the
tutorial, +2 points (up to 6 points maximum)
- 4 points: Eclipse
(awarded according to attendance records for class 1)
- 8 points: Modular Pascal's Triangle
- 2 points: Pair Programming Summary includes definition and reasonable
summary description