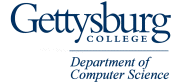 |
CS 112 - Introduction to Computer Science II
Homework #13, Homework #14 |
HW#13 Due Date: At the beginning of class 39.HW#14
Due Date: Friday at the beginning of class 42.
NOTE: This work is to be done in pairs. I strongly recommend the
practice of Pair
Programming described simply
here. Although team members are permitted to divide
work, each team member should be able to informally present all work of
his/her teammate.
Design Project: Interactive Fiction
In the coming two weeks, you will design a simplified interactive fiction
game using classes IFGame.java, Location.java, Item.java. (Inner
class definitions are permitted, but these files, README, and demo.txt, will be
the only files collected and/or compiled.)
What is Interactive Fiction?
Interactive fiction (formerly known as
"text adventures"; similar to multi-user
dungeons/domains (MUDs)) is a form of entertainment in which a user interacts
with and learns about virtual world, usually to achieve a goal. We
will restrict our attention to the genre formerly known as text adventures
where all interaction with the world is through a text-based interface.
Browse the following links for a quick introduction to the world of interactive
fiction:
Specification:
Your interactive fiction system will feature at least six
(eventually) reachable locations, two obstacles (objects which prevent some action,
including movement), two items which, when used with the obstacles (or other
objects), will
allow the player to overcome the obstacles, a goal (explicit or implicit), and
an indication when that goal has been reached. Your interactive fiction
system should behave similar to the ones featured above, in that:
-
the game is turn-based,
-
each turn, the user's environment is described (preferably a brief description
unless the user has just arrived for the first time or typed "look" as
a command),
-
the user can enter simple case-insensitive commands including (but not
necessarily limited to) the minimal subset below:
-
(i)nventory (allowed abbreviation in parentheses)
-
(n)orth, (s)outh, (e)ast, (w)est, (u)p, (d)own (optionally preceded by
the word "go")
-
look - describe environment
-
e(x)amine <object> - give detailed description of an object
-
get/take <object>
-
drop <object>
-
use <object> with <object> (e.g. "use axe with tree". You can also
include intuitive option "chop tree".)
-
restart
-
quit
- if the command cannot be executed, a reasonable response is given, e.g.
(and these need not be your responses)
Helpful Suggestions (not Requirements):
- Feel free to add helpful fields to your class definitions
in ways that support modeling your story. One addition I've found
particularly useful is to add a public Set<Object> attributes field to the
Item class. If you want to designate some Items as gettable (i.e.
obtainable, e.g. key) or not gettable (e.g. door), you can simply add the
String "gettable" to the attributes of gettable Items and check for them
when processing a get command. Similarly, a door Item may have
attributes "door", "closed", and "locked". In short, you can encode and
model rich game state information by adding additional fields to the
appropriate classes.
- Feel free to add helper methods to your class definitions
in order to reduce redundancy. In one IF demo I created which had
multiple doors (including multiple doors in the same location), I found it
beneficial to have a small bit of code that disambiguated door references
for various commands.
- If you're willing to learn more through this experience,
study Java regular expressions (i.e. Regex, see also
text Appendix H). Then you'll find it easier to build
flexible patterns for parsing commands. For example, without
expressions, I might parse a String command allowing a limited number of
player attempts to slide a piece of paper under a door as
"command.equals("slide paper under door") || command.equals("put paper under
door") || ...". However, with the flexibility of pattern matching made
possible with regular expressions, I could easily enable more flexible
player expressions with something like "command.matches("(use|push|put|slide|place)
paper (under|underneath|with|beneath) (cell )?door")" succinctly covering 40
different ways of expressing the same action. If you'd like to learn
more about Java regular expressions,
read this summary and play with this
Regex test code.
HW #13 Deliverables Due at Class 39:
-
README file
-
Java class files in a single submission directory (default package),
including an IFGame.java class that starts your IF game in the main method.
- Support of command line option -e (for echo) which causes your program
to print the command the user just entered. This is particularly
useful for making sense of transcripts when the input source is a file:
java IFGame -e < demo.txt > transcript.txt; less transcript.txt
This previous command feeds the contents of the file to your java program
which echoes the input you can't see otherwise. The output of your
program is redirected to an output file transcript.txt. This is then
paged through using the more command.
- A partial working implementation with a text file demo.txt
which has commands which can be fed to standard input to demonstrate your
IF system. Using the prior -e flag, one should be able to see a
demonstration of travel to six locations, the picking up and dropping of two
items at different locations, with inventory and look commands that verify
the correct moving of items. So, the following commands should be
demonstratably working at this stage:
-
(i)nventory (allowed abbreviation in parentheses)
-
(n)orth, (s)outh, (e)ast, (w)est, (u)p, (d)own (optionally preceded by
the word "go")*
-
look - describe environment
-
e(x)amine <object> - give detailed description of an object
-
get/take <object>
-
drop <object>
- quit
*Note: While all location commands should be recognized as such, your world
map does not need to use all of them. If you can travel north, the
following commands should work: "n", "north", "go n", "go north"
- (What remains for HW#14 is more substantive location/item description,
item interaction with "use <object> with <object>" (e.g. "use axe with
tree" which one might accept along with "chop tree"), restart
ability, and interesting
obstacles/puzzles within a mini-plot.)
Rubric: (20 points total)
- 6 points: at least six reachable locations
- 2 points: at least two items one can get/drop in different
locations
- 1 point: direction commands (e.g. "n", "north", "go n", "go north")
-
1 point: inventory command ("i" or "inventory")
- 1 point: look command
- 1 point: examine command ("x <object>" or "examine <object>")
- 1
point: get/take command ("get <object>" or "take <object>")
- 1 point:
drop command ("drop <object>")
- 1 point: quit command
- 1 point: -e
echo option
- 2 points: a command loop that gives reasonable responses
when either (1) forbidden actions, or (2) nonsense is given as input.
-
2 points: plain text demo.txt with a sequence of commands (one per line)
demonstrating the correct functioning of all of the above functionality
HW #14 Deliverables Due at Class 42:
- README file
-
Java class files in a single submission directory (default package),
including an IFGame.java class that starts your IF game in the main method.
-
Support of command line option -e (for echo) as before.
-
A complete working implementation with a text file demo.txt
w
which has commands which can be fed to standard input to demonstrate a complete
playthrough of your
IF game. If there are multiple playthroughs, include a restart command and
have all playthroughs in your demo.txt file.
Rubric: (20 points total)
- 8 points: HW #13 functionality
- 2 points: sufficient location/item descriptions such that a person
could reasonably guess actions that would lead to achieving the game
goal
- 2 points: item interaction, i.e. an ability to take action with at
least two items in order to affect a change in the game environment.
(The items themselves may/may not change as well, e.g.
crafting/building/destroying.)
- 2 points: restart command
- 2 points: existence of and possibility of achieving a game goal
- 2 points: indication to the user that the goal has been achieved
- 2 points: plain text demo.txt with a sequence of commands (one per
line) demonstrating the correct functioning of all HW #13 and HW #14
functionality