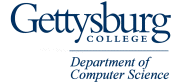 |
CS 112 - Introduction to Computer Science II
Homework #5 |
Due: At the beginning of class 15.NOTE: This work is to be done in pairs. I strongly recommend the
practice of Pair
Programming described simply
here. Although team members are permitted to divide
work, each team member should be able to informally present all work of
his/her teammate.
Exceptional FreeCell
1. Exceptional FreeCell: Revise your code from
Homework #4 to reflect the proper use of exceptions according to
this specification. That is:
- Your playTo methods should throw NullPointerExceptions if given null
parameters. No printed error message is necessary.
- When one calls getTopCard/removeTopCard on an empty CardStack, do not
return null. Rather simply call peek/pop, respectively, and let an
EmptyStackException be thrown. Do not attempt to guard against the stack
exception (thrown for you) by returning null. Simply return the result
of the peek/pop, respectively.
- When one calls canPlayFrom on an empty CardStack throw an
IllegalPlayException with the message "You cannot play from an empty stack."
(which in the gameplay loop causes the printing of the message "Illegal play: You cannot play from an empty stack.").
- When one attempts to make an illegal play, throw an Exception class
extension that you are to define: IllegalPlayException(String message).
When this exception is caught, a specified error message (below) is printed
(preceded by "Illegal play: ") and the attempted play is
ignored. "Illegal play: " is not included in the IllegalPlayException
message, but is added when the exception is caught in Freecell.java.
- When one enters a non-integer, the Scanner itself will throw a specific
exception. (See the Java Package Documentation for Scanner's nextInt
method to see which one!) Gracefully recover from this exception with
an appropriate message (below). (Read the rest of the line before resuming.
For example, the input line "forty 2" would throw an exception on "forty",
but we wouldn't want to start fresh with the " 2" as the first stack of the
next input. Method nextLine() of Scanner will read to the next line.)
- Out of range, or duplicated stack numbers are handled as
IllegalPlayExceptions
giving the appropriate message below.
This transcript using game seed 2 illustrates the helpful error
messages that can be communicated through exception handling. (Note: The plays
shown are the input and are not printed on the output.)
Specified error messages:
- Source and destination card stacks must be entered as integers (1-16).
- Illegal play: Illegal stack number. Stacks are numbered 1-16.
- Illegal play: Source and destination stacks must be different.
- Illegal play: You cannot play from an empty stack.
- Illegal play: The first foundation card must be an Ace.
- Illegal play: Plays to a foundation must match suit.
- Illegal play: Plays to a foundation must have the next increasing rank.
- Illegal play: That card stack cannot be played from. (thrown from
Deck and Foundation canPlayFrom methods)
- Illegal play: Cells may only contain a single card.
- Illegal play: Plays to a cascade must alternate in suit color.
- Illegal play: Plays to a cascade must have the next decreasing rank.
Notes:
- An attempted play to a foundation/cascade may be illegal for multiple
reasons. Prioritize reporting suit/suit color errors over rank errors.
Examples of code modification:
- An IllegalPlayException is a trivial extension of the Exception class.
- An attempted play that would cause a peek/pop from an empty stack should result in throwing a new IllegalPlayException with an approapriate message above.
- Illegal plays likewise no longer simply return false to a boolean type method. Rather, it should result in throwing a new IllegalPlayException with an appropriate message above. Accordingly look for where you return false in your previous card stack classes, have the method declared with "throws IllegalPlayException", and replace false returns with the throwing of a new IllegalPlayException
with an appropriate specified message.
- The FreecellGame class play method should now also throw an IllegalPlayException with messages as given in the 2nd and 3rd bullets above.
- In Freecell.java, one must surround the call to the FreecellGame play method with a try-catch block that catches an IllegalPlayException and prints "Illegal play: " and the message of the exception on a single line.
Rubric: (20 points total)