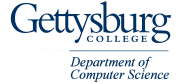 |
CS 341 - Principles of Programming Languages
Fourth Hour Homework: Language Design |
Due: Various dates. See below.
Note: This work is to be done in pairs. Each pair will
submit one assignment. Although you may divide the work, both team members
should be able to present/describe their partner's work upon request.
Language Design Phase 1
Due: at the beginning of class 15
As our Fourth Hour project for the semester, you will be designing your own
high level programming language and implementing an interpreter for it using
JavaCC. As a first step you need to work through your design thoughts
concretely by writing example implementations using your proposed language.
Implement all 6 algorithms of Homework #1 using your
proposed language. You should at this point have working examples in a
number of languages, so the challenge should not be algorithmic. Rather,
you will be focused on the design of your language.
NOTE: It's important that your language not merely make the 6 algorithms trivial by including single commands that solve those problems, e.g. "process_binarysearch_tree_int_commands_from_stdio();". Think about best syntax and semantics from a tokenizing, parsing, and general purpose programming perspective.
To submit this homework, email (with subject "submit341 ld1") or physically submit your code on paper to me directly.
Language Design Phase 2
at the beginning of class 20
- Create source files in your language for the maximum and factorial
problems of Homework #1. Call these maximum.txt
and factorial.txt.
- Implement an interpreter for your language that can, using the JavaCC
command "javacc MyLang.jj" and the java compiler command "javac
MyLang.java", create a Java executable that can interpret a source code file
<src> and input file <in> as follows: "java MyLang <src> <
<in>", where <src> is one of the source code files of step 1.
- Submit your interpreter and source code from the directory containing
them using the command "submit341 ld2".
Note: As you seek to define operator precedence in your grammar, you may find
my MiniJava project
"Precedence Hierarchy Process" documentation helpful for modeling after
Java's operator precedence.
Language Design Phase 3
Due: at the beginning of class 23
- Create additional source files in your language for the unique and
quicksort problems of Homework #1. Call these
unique.txt and quicksort.txt.
- Implement an interpreter for your language that can, using the JavaCC
command "javacc MyLang.jj" and the java compiler command "javac
MyLang.java", create a Java executable that can interpret a source code file
<src> and input file <in> as follows: "java MyLang <src> <
<in>", where <src> is one of the source code files of step 1. All
source code files of phase 2 and phase 3 should function correctly.
- Submit your interpreter and source code from the directory containing
them using the command "submit341 ld3".
Language Design Phase 4
Due: at the beginning of class 26
- Create additional source files in your language for the binary search
tree and breadth-first search problems of Homework #1.
Call these binary-search-tree.txt and breadth-first-search.txt.
- Implement an interpreter for your language that can, using the JavaCC
command "javacc MyLang.jj" and the java compiler command "javac
MyLang.java", create a Java executable that can interpret a source code file
<src> and input file <in> as follows: "java MyLang <src> <
<in>", where <src> is one of the source code files of step 1. All
source code files of phases 2, 3, and 4 should function correctly.
- Submit your interpreter and source code from the directory containing
them using the command "submit341 ld4".
Note that, for phases 3 and 4, you'll include the source code for problems of
previous phases.
Presentation
Prepare a 10-minute-maximum presentation of your programming language to share with the class. What to include:
- An overview of your code's syntax and semantics. I would limit it to a couple code examples, e.g. maximum (simple) and binary search tree (complex). In the complex example, focus on language features (not teaching BST algorithms).
- Demonstrate the execution of the code on simple small examples. For example, the maximum of three integers 12, 23, and -42, and the BST command processing of 5 or so command-key pairs.
- Feel free to show your latest feedback.txt file.
- Sample questions to answer: Which language is your language most like? What do you like best about it? What was most challenging in the design?
Presentations should be more than 5 minutes.