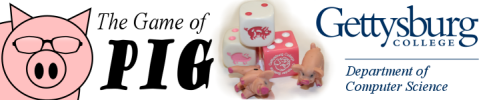
Pig Game GUI
Pig is a folk jeopardy dice game with simple rules: Two players race to reach 100 points. Each turn, a
player repeatedly rolls a die until either a 1 ("pig") is rolled or the player holds and
scores the sum of the rolls (i.e. the turn total). At any time during a
player's turn, the player is faced with two decisions:
- roll - If the player rolls a
- 1: the player scores nothing and it becomes the opponent's
turn.
- 2 - 6: the number is added to the player's turn total and the
player's turn continues.
- hold - The turn total is added to the player's score and it
becomes the opponent's turn.
Problem: Implement a GUI for the game of Pig where the user plays
a succession of games against a computer player. A brief summary of
software requirements follows.
Overview
Your goal is to create a simple-yet-fun GUI implementation of the game of
Pig. The user is the starting player of the first game. The
starting player alternates with successive games. The computer plays a
"Keep Pace and End Race" policy as follows: Let the hold value be 21 + round((user score - computer score) / 8). If either player has 71 points
or more, or if the turn total is less than the hold value, then roll.
Otherwise, hold.
Resources
Image files are supplied in
PNG and JPEG formats.
Graphical User Interface Components
Main application frame titled "Pig", terminating program on close, and
containing:
- "Your Score:" label - fixed text label
- Your score value label - should update to reflect changes in user score
- "My Score:" label - fixed text label
- My score value label - should update to reflect changes in computer
score
- "Turn Total:" label - fixed text label
- Turn total value label - should update to reflect changes in turn total
- Die panel - area for displaying 100-by-100 die images, and roll/hold
images
- "Roll" button - enabled during user turn to trigger Roll Action
- "Hold" button - enabled during user turn to trigger Hold Action
- Pop-up window with:
- Game over text label - provides winner, final score, and prompts
user with new game decision.
- "New Game" button - triggers New Game Action
- "Quit" button - exits application
For the most part, layout is not specified. However, the related
label-value label pairs should be horizontally adjacent with the label to the
left of the value label.
Functional Specification
Initialization
- Create and layout all GUI components with the exception of the pop-up
window.
- Set both scores and turn total to 0.
- Display the "roll" image in the die panel.
- Set the starting player to the user.
- Set the current player to the user.
Roll Button
- Enable roll button during user turn.
- On roll button press, trigger Roll Action.
Roll Action
- Generate a random number r in the range [1, 6].
- Display the "die<r>" image in the die panel.
- If r is 1 ("pig"), set the turn total to 0, and trigger Change
Turn Action.
- Otherwise, add r to the turn total. If the current player score plus the
turn total is greater than or equal to the goal score of 100, trigger Hold
Action.
Hold Button
- Enable hold button during user turn.
- On hold button press, trigger Hold Action.
Hold Action
- Display the "hold" image in the die panel.
- Add the turn total to the current player's score.
- Set the turn total to 0.
- If the current player's score is greater than or equal to the goal score
of 100, trigger End Game Action.
- Otherwise, trigger Change Turn Action.
Change Turn Action
- Change the current player.
- Enable/disable roll and hold buttons if the user/computer is the current
player, respectively.
- If it is the computer's turn, begin the Computer Turn.
Computer Turn
- Note: In
many cases, a separate thread is advisable for the computer player to
prevent GUI updates from blocking.
- While the computer is the current player:
- Delay for approximately 1 second.
- Compute a hold value of 21 + round((user score - computer score) / 8).
- If either player has 71 points
or more, or if the turn total is less than the hold value, then trigger Roll
Action. Otherwise, trigger Hold Action.
End Game Action
- Create an display a popup window with:
- Game over text label - If the user has won, display "You win <user
score> to <computer score>. Would you like to play again?" If the
computer has won, display "I win <computer score> to <user score>. Would
you like to play again?".
- "New Game" button
- "Quit" button
- When the "New Game" button or "Quit" button is pressed, the
popup window
closes.
- If the "New Game" button is pressed:
- Set both scores and the turn total to 0.
- Change the starting player.
- If the current player is different than the starting player, trigger the
Change Turn Action.
- If it is the computer's turn, begin the Computer Turn. Otherwise,
display the "roll" image in the die panel.
- If the "Quit" button is pressed, the application terminates, closing the
main application frame.
Extra Exercises:
1. Use an alternative computer play policy. Let i be the player's score, j
be the opponent's score, and k be the current turn total.
Some alternative play policies:
- Hold at 25 or goal - Hold at the lesser of 25 and 100 - i.
- 4 Scoring Turns - Let t be the number of turns in which a
player has held so far. Hold at floor((100 - i)/(4 - t)).
- Score Base, Keep Pace, and End Race - If i >= 69 or j
>= 69, roll for the goal. Otherwise, hold at the greater of 19 and
j - 14.
2. Compute win statistics (e.g. games won, games lost, percentage won) and
display these at game end.
3. Extend design to three or more user/computer players.
4. (Advanced Project) Extend design to three or more user/computer players
over a network, using a client-server model.
Todd Neller