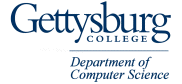 |
CS 111 - Introduction to Computer Science I
Pre-Class and In-Class Activities |
Due: The work before each
class is due at the beginning of the class as indicated below.
Note: You will need to eventually be working on a machine with Eclipse, a terminal window, and a web browser. For the first class, only a Linux terminal window with SSH is needed. (In person, we have such machines in our lab. When we start remote, we need to get a way for you to connect to these machines remotely.)
Instructions for setting up your machine for remote work are at the page Tools for Working Remotely. If you would have difficulty accessing/configuring such a machine please contact me.
- CS 111 home page
-
Tools for Working Remotely
-
My Office Hours are listed on the course information page.
-
Student Help Hours Sunday through Thursday 7-9PM Eastern in Glatfelter 207 (starting week 2)
-
Remember to do all readings and assigned video exercises before each assigned class. If you cannot access your textbook, one can generally find a 10th edition Liang text by searching "liang introduction to java programming comprehensive version 10th edition pdf".
-
You are expected to read your Gettysburg email at least once a day. Required CS Colloquia will be announced via email. You are required to attend two during the semester. Also, assignment clarifications and other important information may be shared periodically through the class mailing list.
-
Before class 1 ():
- Assigned readings:
- OPTIONAL: Install a free SSH client program on your computer. Instructions for this and how to install all necessary course software are on our Tools for Working Remotely page. For PC, we recommend MobaXTerm. For Mac, there is already a single built-in command you can enter in your Terminal window, so please learn how to open a (Linux) Terminal window and install XQuartz (step 2). It is not necessary to set up all software in advance (e.g. Java, Eclipse). That will be one of our tasks for the week, and we will offer lots of support. On Monday, we will just be wanting to see that you can log into our system directly and change your password on our system to match your Gettysburg password or be something at least more intuitive. If your account with us is new, you should have received your password before class 1, and we will walk you through the login procedure in class.
- Class 1 ():
- Welcome and introduction
- Logging onto our system
- Changing passwords
- Course overview
- Before Class 2 ():
- Class 2 ():
- Java compilation and interpretation
- Hello.java ("Hello, world!") from the command line with a text editor.
- Before Class 3 ():
- Assigned readings
- Seek to complete all of HW1 except for questions 3 (done in-class on Friday) and 4 (a simple modification of 3 to practice the process).
- Complete any remaining Tools for Working Remotely for your personal computer.
- Class 3 ():
- Hello.java ("Hello, world!") via Eclipse
- Reference: Eclipse Hello World Tutorial for Linux. The "Open Eclipse:" first 2 steps will be different for a PC or Mac, i.e. just start the application.
- Review of HW1 (due Monday in class) and coming programming quizzes
- Before Class 4 ():
- Assigned readings
- Gather all necessary HW1 files (Hello.java, HelloMe.java, README) in one directory (folder) in your CS account.
- Ch2Fun.java video part 1 - Program along and have this portion of Ch2Fun.java implemented before class 4.
- Class 4 ():
- Homework #1 submission
- Homework #2 overview
- Weekly Rhythm
- Challenge problems (as time allows):
- Prompt the user for two integers and print their floating-point average.
- Choose from text exercises 2.2-2.4.
- Before Class 5 ():
- Class 5 ():
- Programming Quiz (short)
- ContinuedFraction.java - continued fraction example
- Before Class 6 ():
- Class 6 ():
- RuleOf72.java - Rule of 72 Software Design Example
- Homework #3 overview
- Begin HW3 (as time allows)
- Before Class 7 ():
- Class 7 ():
- SubtractionGame.java (example transcript below)
- Let maxPieces be the maximum number of pieces a player can take.
- Let numPieces be the current number of pieces input by the user.
- Let numRemove be the remainder when numPieces is divided by (maxPieces + 1).
- If the player can take all remaining pieces, print "Remove all.". Otherwise, print "Remove #." where # is numRemove, unless it's illegal (0), in which case, simply print "Remove 1.".
Subtraction Game: Starting with some number of pieces,
two players take turns removing 1-6 pieces at a time.
The player that removes the last piece wins.
How many pieces remain? 30
Remove 2.
Before Class 8 ():
Class 8 ():
- Programming Quiz 2
- RockPaperScissors.java (see below)
In-class exercise: Rock-Paper-Scissors
(text exercise 3.17 modified) Call your program
RockPaperScissors.java.
Input: an integer 0, 1, or 2, representing a play of "rock", "paper",
or "scissors", respectively.
Output:
- Before the input: a prompt "Please enter 0 (rock), 1 (paper), or
2 (scissors): "
- After the input:
- The computer randomly chooses an integer to represent it's play.
- Print "I played <play>." where <play> is
"rock"/"paper"/"scissors" depending on the computer's randomly chosen
integer.
- Print "You win."/"You lose."/"Draw." based on the following rules:
- Rock wins against scissors.
- Scissors wins against paper.
- Paper wins against rock.
- Players that choose the same play draw (i.e. tie).
Hint: At no point should you have an if-else chain for 9 cases.
Each of the last two print statements should require at most 3 cases
each. Let the user's play be p1 and the computer's play be p2.
Consider what value you get if you compute (p1 - p2 + 3) % 3. How
does the result of this computation map to the win/lose/draw
cases? Why does this work? Why wouldn't the expression (p1 - p2) % 3 suffice for our three cases?
Example transcripts (input underlined):
Please enter 0 (rock), 1 (paper), or 2 (scissors): 0
I played paper.
You lose.
Please enter 0 (rock), 1 (paper), or 2 (scissors): 1
I played rock.
You win.
Please enter 0 (rock), 1 (paper), or 2 (scissors): 2
I played scissors.
Draw.
Before Class 9 ():
Class 9 ():
- Homework #4 Overview
- Number guessing game turn exercise (below)
In-class exercise: Number Guessing Game Turn
Call your program
NumberGuess.java.
Input: an integer guess of the program's secret number
Output:
- A line indicating the range of the computer's random secret number from 1 through
maxSecret
, an internal variable. If maxSecret
is 10, then we print "I'm thinking of a number from 1-10."
- A prompt for the user requesting their guess. Without a newline print "Your guess? ".
- A line with an appropriate response to the user guess (correct/higher/lower) and what the player can deduce. Examples of all output cases are in the transcripts below.
I'm thinking of a number from 1-10.
Your guess? 5
Lower. Thus, my number is at least 1 and at most 4.
I'm thinking of a number from 1-100.
Your guess? 50
Higher. Thus, my number is at least 51 and at most 100.
I'm thinking of a number from 1-5.
Your guess? 3
Correct!
Before Class 10 ():
Class 10 ():
- Pos2DUpdate.java (see below)
In-class exercise: 2D Position Update
Call your program
Pos2DUpdate.java. In this exercise, you'll imagine yourself as a video game designer. In a 2D game, imagine that you are updating the position of a game object centered at (x, y). To do an update to the position, you'll need to know its speed, direction, and the time step over which the update will take place. If you don't know the trigonometry needed for this computation, search for it online. All values are assumed to be of double type.
Input: (x, y) position, speed (1/seconds), direction (radians), time step (seconds)
Output:
- Prompts for each double input: "x: ", "y: ", "speed (1/seconds): ", "direction (radians): ", "time step (seconds): "
- A line consisting of "New position: (#, #)" where the two numbers are the new x and y position after one time step, assuming constant speed and direction.
x: 0
y: 0
speed (1/seconds): 1
direction (radians): 0
time step (seconds): .1
New position: (0.100000, 0.000000)
x: 1
y: 1
speed (1/seconds): 2
direction (radians): 3.14159265358
time step (seconds): .1
New position: (0.800000, 1.000000)
x: 1
y: 2
speed (1/seconds): 3
direction (radians): 2
time step (seconds): .01
New position: (0.987516, 2.027279)
Before Class 11 ():
Class 11 ():
- Programming Quiz 3
- Sort3Strings.java (see below)
In-class exercise: Sorting Three Strings
Call your program
Sort3Strings.java. In this exercise, you'll input three Strings and print them out in sorted order. In this context, sorted order is "lexicographical order", sorted as we would alphabetically except that we're sorting according to Unicode values, so all uppercase characters would come before lowercase characters, etc. Extra challenge: Modify your program to be case insensitive when doing lexicographic sorting.
Input: three strings, each read as a whole line
Output:
- Prompts for each String: "String 1? ", "String 2? ", "String 3? "
- the same three input strings, one per line, ordered lexicographically.
String 1? Testing
String 2? one
String 3? two
Testing
one
two
String 1? c
String 2? b
String 3? a
a
b
c
String 1? A
String 2? 1
String 3? a
1
A
a
Before Class 12 ():
Class 12 ():
- Homework #5 overview
- LogisticChaos.java: Loop preview with mathematical exploration.
- Guess.java: Guess the secret number game pseudocode and commenting.
Before Class 13 ():
Class 13 ():
- Guess.java: Implement guessing game pseudocode.
Before Class 14 ():
Class 14 ():
- Programming Quiz 4
- Guess2.java: Have the computer guess your secret number.
Before Class 15 ():
Class 15 ():
Include this code snippet:
// https://www.space.com/mars-rover-perseverance-landing-explained
double height = 2100; // meters where the sky crane takes over with thrusters
double velocity = -320000.0 / 3600; // meters per second initially
double maxHeight = 20; // meters max height for lowering Perseverance
double maxVelocity = -2700.0 / 3600; // meters per second max speed for lowering Perseverence
double accelGravity = -3.72076; // m s^-2 https://en.wikipedia.org/wiki/Gravity_of_Mars
double maxThrust = 8 * 3558.58; // kg m s^-2 max Newtons of thrust (https://www.wired.com/story/how-to-watch-nasa-launch-its-new-perseverance-mars-rover/)
// 8 thrusters each capable of 800 lbs. of thrust?
double massSkycrane = 1370; // kg https://weebau.com/satplan/msl.htm (includes Perseverance?)
// double massPerseverance = 899; // kg https://en.wikipedia.org/wiki/Mars_Science_Laboratory#Spacecraft
double maxDecel = maxThrust / massSkycrane; // ms^-2
System.out.println("Max Deceleration: " + maxDecel);
double maxTime = 60; //https://www.nbclosangeles.com/news/local/timeline-nasa-mars-perseverance-rover-landing-seven-minutes-terror-jpl-space/2528737/
double deltaT = .1; // arbitrary unit of time step for simulation
double time = 0;
Before Class 16 ():
Class 16 ():
- Complete Skycrane.java
- NewtonsMethod.java: Class exercise for development of Newton's Method. Also relevant: central difference approximation of a derivative. Use static methods (f, fPrime, newton, etc.) to find roots of f using Newton's Method. Try it with f(x) = x3 - 2x (video part 1 (24:12), part 2 (12:44))
Before Class 17 ():
Class 17 ():
- Programming Quiz 5 (30 minutes)
- Continued NewtonsMethod.java:
- Complete Netwon's Method if you haven't already.
- See what happens for x0 = 0, 1 for f(x) = x3 - 2x + 2. Devise a solution to allow termination of Newton's Method in such circumstances.
- Create another method for iteratively searching for all 4 real roots, and test it on f(x) = x4 - 929.9 x3 - 853844.0 x2 - 1.9589×106 x + 24.1729
Before Class 18 ():
Class 18 ():
- Homework #7 Overview
- Quiz practice for Midterm preparation
Before Class 19 ():
Class 19 ():
- CellularAutomata.java - Binary Numbers, Single Dimensional Arrays, and Cellular Automata
Before Class 20 ():
Class 20 ():
- CellularAutomata.java completion
- Programming Quiz 6 (30 minutes)
Before Class 21 ():
Class 21 ():
Before Class 22 ():
Class 22 ():
Before Class 23 ():
Class 23 ():
Before Class 24 ():
Class 24 ():
Before Class 25 ():
Class 25 ():
Before Class 26 ():
Class 26 ():
- Programming Quiz 8 (30 min.)
- Kattis exercises of your choice.
Before Class 27 ():
Class 27 ():
Before Class 28 ():
Class 28 ():
- Polynomial.java - an example in test-driven, object-oriented development
Before Class 29 ():
Class 29 ():
- Programming Quiz #9 (30 min.)
- Polynomial.java (cont.)
- Kattis exercises of your choice.
Before Class 30 ():
Class 30 ():
Before Class 31 ():
Class 31 ():
Before Class 32 ():
Class 32 ():
Before Class 33 ():
Class 33 ():
Before Class 34 ():
Class 34 ():
- RationalApprox.java (cont. if needed)
- ArrayList practice: ArrayListSort.java - Read a list of integers (number of integers unknown) from the standard input, sort them, and print them to the standard output. Practice with Linux command line redirection of input ("< inputFilename > outputFilename").
- Kattis exercises of your choice.
Before Class 35 ():
Class 35 ():
Before Class 36 ():
Class 36 ():
Before Class 37 ():
Class 37 ():
Before Class 38 ():
Class 38 ():
Before Class 39 ():
Class 39 ():
- Overview of Homework #14 implementation of Breakthrough with an "undo" capability using a Stack and a deep Cloneable BreakthroughState.
- Programming practice
Before Class 40 ():
Class 40 ():
Before Class 41 ():
Class 41 ():
Before 42 ():
Class 42 ():